CRUD with Python and DynamoDB: A Step-by-Step Guide
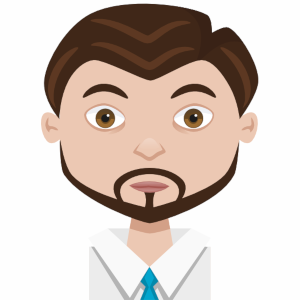
CRUD stands for Create, Read, Update, and Delete. These are the basic operations that most data-driven applications perform on their data. In this project, we will create a CRUD application using Python and DynamoDB. DynamoDB is a NoSQL database provided by Amazon Web Services that offers a scalable and flexible solution for storing and retrieving data.
For this project, we will use the Boto3 library for Python, the official SDK for AWS. Boto3 makes it easy to interact with AWS services like DynamoDB using Python code. Let’s get started!
Step 1: Setting up your environment
Before we start coding, we need to set up our environment. Here are the steps to follow:
- Create an AWS account if you don’t already have one.
- Create an IAM user with programmatic access and attach the AmazonDynamoDBFullAccess policy.
- Install the Boto3 library by running
pip install boto3
in your terminal. - Create a DynamoDB table with a primary key called
id
of typeString
.
Step 2: Writing the code – CRUD with Python and DynamoDB
Now that our environment is set up let’s start writing some code! We’ll create a Python file called crud.py
and start with the necessary imports:
import boto3
from boto3.dynamodb.conditions import Key, Attr
Next, we’ll create a DynamoDB
object using our credentials:
dynamodb = boto3.resource('dynamodb',
region_name='us-east-1',
aws_access_key_id='YOUR_ACCESS_KEY',
aws_secret_access_key='YOUR_SECRET_KEY')
Make sure to replace YOUR_ACCESS_KEY and YOUR_SECRET_KEY with your actual IAM user credentials.
Now, let’s define our CRUD
functions one by one:
How to Boost Your AWS Skills
Don’t miss out on the opportunity to take your cloud computing skills to the next level with our free AWS Learning Kit! Whether you’re an aspiring cloud professional or a seasoned veteran, AWS is a must-know platform for any successful cloud career.
By downloading our Learning Kit, you’ll gain access to comprehensive resources, including tutorials, case studies, and practice exams, that will prepare you for AWS certification and equip you with the knowledge and skills needed to succeed in the cloud industry. Start investing in your future today and download our AWS Learning Kit for free!
Create
def create_item(table_name, item):
table = dynamodb.Table(table_name)
response = table.put_item(Item=item)
return response
This function takes the name of the DynamoDB table and an item to insert. We create a Table object using the table name and then call the put_item method to insert the item into the table. Finally, we return the response from the put_item method.
Read
def get_item(table_name, item_id):
table = dynamodb.Table(table_name)
response = table.get_item(Key={'id': item_id})
return response.get('Item')
This function takes the name of the DynamoDB table and the item ID to retrieve. We create a Table object using the table name and then call the get_item method with the Key parameter set to the ID of the item we want to retrieve. Finally, we return the Item as an object from the response.
Update
def update_item(table_name, item_id, update_expression, expression_values):
table = dynamodb.Table(table_name)
response = table.update_item(
Key={'id': item_id},
UpdateExpression=update_expression,
ExpressionAttributeValues=expression_values,
ReturnValues="UPDATED_NEW"
)
return response.get('Attributes')
This function takes the name of the DynamoDB table, the item’s ID to update, an update expression, and expression values to substitute into the expression. We create a Table object using the table name, and then call the update_item method with the Key parameter set to the ID of the item we want to update, the UpdateExpression parameter set to the update expression, and the ExpressionAttributeValues parameter set to the expression values.
The ReturnValues the parameter is set to "UPDATED_NEW"
return only the updated attributes of the item. Finally, we return the updated attributes from the response.
Delete
def delete_item(table_name, item_id):
table = dynamodb.Table(table_name)
response = table.delete_item(Key={'id': item_id})
return response
This function takes the name of the DynamoDB table and the item ID to delete. We create a Table object using the table name, and then call the delete_item method with the Key parameter set to the ID of the item we want to delete. Finally, we return the response from the delete_item method.
Step 3: Testing the code
Now that we’ve written our CRUD
functions, let’s test them out! Here’s an example of how to use the functions:
# Define the name of your DynamoDB table
TABLE_NAME = 'my-table-name'
# Define an item to insert
item = {
'id': '1',
'name': 'John',
'age': 30
}
# Create the item
response = create_item(TABLE_NAME, item)
print(response)
# Retrieve the item
item = get_item(TABLE_NAME, '1')
print(item)
# Update the item
update_expression = 'set #a = :val1'
expression_values = {':val1': 35}
response = update_item(TABLE_NAME, '1', update_expression, expression_values)
print(response)
# Delete the item
response = delete_item(TABLE_NAME, '1')
print(response)
This code creates an item with an ID of 1, a name of ‘John’, and the age of 30. We then insert the item into the DynamoDB table using the create_item function, retrieve it using the get_item function, update it to set the age to 35 using the update_item function, and finally, delete it using the delete_item function.
Best practices
Here are some best practices to follow when using DynamoDB:
- Choose the proper partition key to ensure an even data distribution across partitions.
- Use batch operations to improve performance when working with multiple items.
- Use the
ProjectionExpression
parameter to retrieve only the attributes you need. - Use conditional expressions to ensure atomic updates and deletes.
- Use DynamoDB streams to process changes to your data in real time.
That’s it! I hope this project helps you start building a CRUD application using Python and DynamoDB.
Comments